鸿蒙学习基础环境搭建、基本的语法、以及构建视图的基本语法(一)
敬礼,感谢收看!
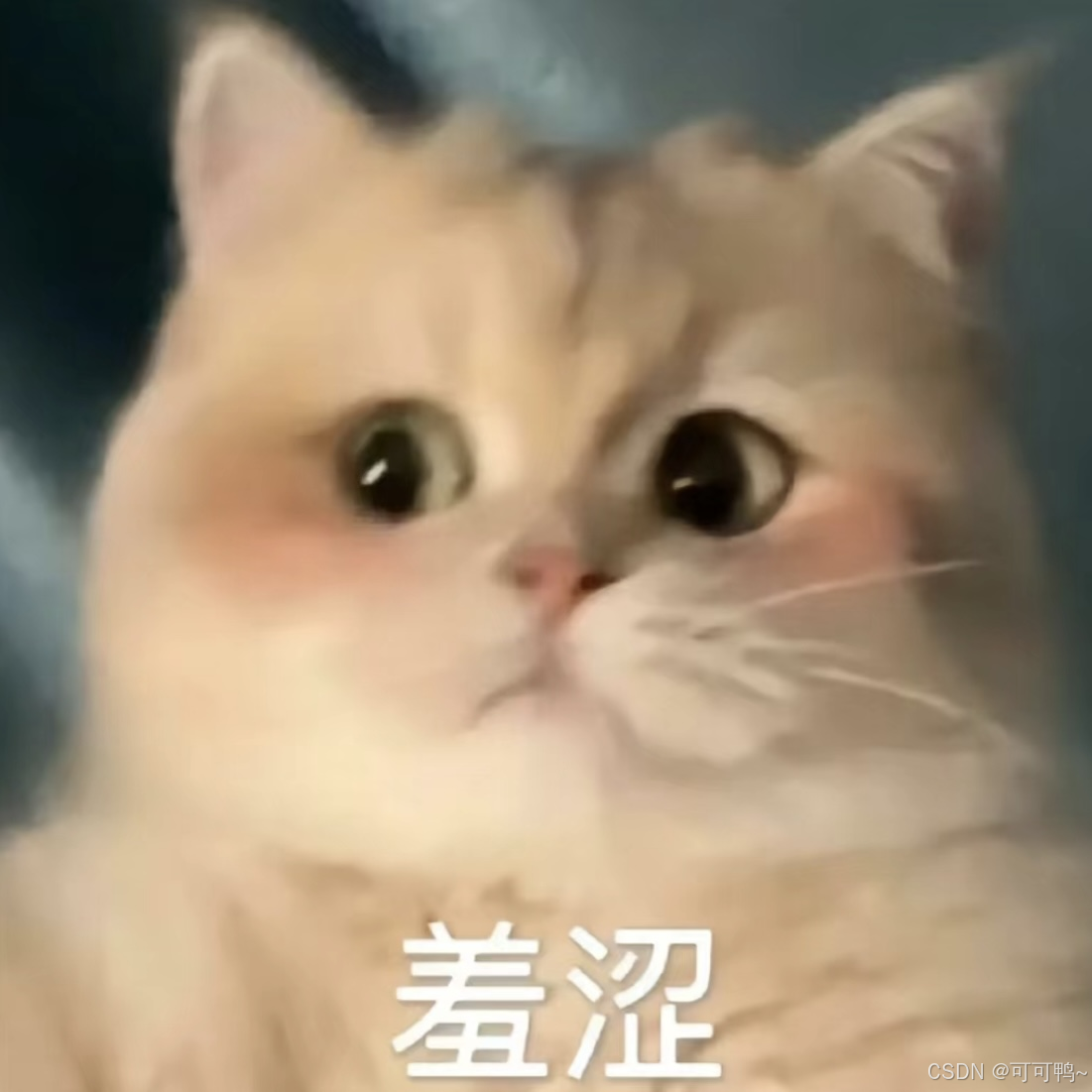
一、基础环境搭建
1.下载鸿蒙开发工具
DevEco Studio 5.0.1 Release下载
1.下载直接next傻瓜安装就可以
2.下载完毕到setting中找到sdk编辑
3.下载完毕在重新启动一下,点击diagnose诊断环境
4.要是都没问题就可以创建项目了,点击Projects,再点击Create Project。
二、创建第一个项目
1.点击新建项目选第一个模板就可以
2.点击预览器,点击运行箭头预览项目
3.在build上面输出第一个语句控制台查看输出结果
console.log("我说",'helloWord')
三、ARKTs基本的语法
1.打印
console.log("我说",'helloWord')
2.变量存储和修改
// console.log('消息说明', '打印的内容')
// console.log('我说', '学鸿蒙')
// 变量的存储和修改(string number boolean)
// 1. 变量存储
// 1.1 字符串 string 类型
// 注意点1:字符串需要用引号引起来(单引双引号)'字符串' "字符串"
// 注意点2:存储的时候,后面的存的内容,需要和前面的类型对应
let title: string = '巨无霸汉堡'
console.log('字符串title', title)
// 1.2 数字 number 类型
let age: number = 18
console.log('年纪age', age)
// 1.3 布尔 boolean 类型(true真,false假)
let isLogin: boolean = false
console.log('是否登录成功', isLogin)
// 2. 变量的修改
age = 40
console.log('年纪age', age)
// 常量
const PI: number = 3.14
const companyName: string = '华为'
console.log('公司名:', companyName)
console.log('PI:', PI)
// 命名规则:
// ① 只能包含数字、字母、下划线、$,不能以数字开头 -- 重点
// let num-1: number = 11 // 错误 特殊字符
// let num_1: number = 11 // 正确
// let num$: number = 200 // 正确
// let num^ : number = 200 // 错误 特殊字符
//
// let num1: number = 11 // 正确
// let 1num: number = 22 // 错误 以数字开头
// ② 不能使用内置关键字或保留字 (比如 let、const)
// let let: number = 100
// let const: string = 'abc'
// ③ 严格区分大小写
// let myName: string = '吴彦祖'
// // let myName: string = '邓超'
// let MyName: string = '刘德华'
// console.log('myName', myName)
// console.log('MyName', MyName)
struct Index {
message: string = 'Hello World';
build() {
Row() {
Column() {
Text(this.message)
.fontSize(50)
.fontWeight(FontWeight.Bold)
}
.width('100%')
}
.height('100%')
}
}
3.数组
// 学生数组
// 语法:
// let 数组名: 类型[] = [数据1, 数据2, 数据3, ... ]
// 数组中存储的每个数据,都有自己的编号,编号从0开始(索引)
let names: string[] = ['刘亦菲', '杨颖', '杨幂', '刘诗诗', '伍佰']
console.log('数组names', names)
// 利用有序的编号(索引) 取数组的数据
// 取数据:数组名[索引]
console.log('取到了', names[3])
struct Index {
message: string = 'Hello World';
build() {
Row() {
Column() {
Text(this.message)
.fontSize(50)
.fontWeight(FontWeight.Bold)
}
.width('100%')
}
.height('100%')
}
}
4.方法
// console.log('五角星', '☆')
// console.log('五角星', '☆☆')
// console.log('五角星', '☆☆☆')
// console.log('五角星', '☆☆☆☆')
// console.log('五角星', '☆☆☆☆☆')
//
// console.log('五角星', '☆')
// console.log('五角星', '☆☆')
// console.log('五角星', '☆☆☆')
// console.log('五角星', '☆☆☆☆')
// console.log('五角星', '☆☆☆☆☆')
//
// console.log('五角星', '☆')
// console.log('五角星', '☆☆')
// console.log('五角星', '☆☆☆')
// console.log('五角星', '☆☆☆☆')
// console.log('五角星', '☆☆☆☆☆')
// 1. 定义函数 (只是定义了函数,但是没有使用,不会执行内部的代码段)
function star() {
console.log('五角星', '☆')
console.log('五角星', '☆☆')
console.log('五角星', '☆☆☆')
console.log('五角星', '☆☆☆☆')
console.log('五角星', '☆☆☆☆☆')
}
// 2. 调用函数(使用函数)
// 函数名()
star()
star()
star()
struct Index {
message: string = 'Hello World';
build() {
Row() {
Column() {
Text(this.message)
.fontSize(50)
.fontWeight(FontWeight.Bold)
}
.width('100%')
}
.height('100%')
}
}
5.箭头函数
// 需求1:
// 用箭头函数,包裹打印五角星的代码,实现多次打印
// console.log('五角星', '☆')
// console.log('五角星', '☆☆')
// console.log('五角星', '☆☆☆')
// console.log('五角星', '☆☆☆☆')
// console.log('五角星', '☆☆☆☆☆')
// let star = () => {
// console.log('五角星', '☆')
// console.log('五角星', '☆☆')
// console.log('五角星', '☆☆☆')
// console.log('五角星', '☆☆☆☆')
// console.log('五角星', '☆☆☆☆☆')
// }
// star()
// star()
// star()
// 需求2:
// 用箭头函数,完成计算
// 传入 价格 和 数量,返回 计算的结果
// 1. 苹果 2元/斤, 买了3斤,多少钱?
// 2. 香蕉 4元/斤, 买了4斤,多少钱?
let buy = (price: number, weight: number) => {
// 1. 计算数据
let result: number = price * weight
// 2. 返回计算的结果
return result
}
let apple: number = buy(2, 3)
console.log('苹果', apple)
let banana: number = buy(4, 4)
console.log('香蕉', banana)
struct Index {
message: string = 'Hello World';
build() {
Row() {
Column() {
Text(this.message)
.fontSize(50)
.fontWeight(FontWeight.Bold)
}
.width('100%')
}
.height('100%')
}
}
4.接口
*interface
*对象 对象就是可以存取多个盒子的容,用于描述物体的特征和行为
*接口
* interface Person{
* name:string
* age:number
* }
* * let ke:Person = {
* name:"可可",
* age:20
*
* }
* 使用 ke.name
* 对象-方法
* interface 接口没名称{
* 方法名称:(参数:类型) => 返回值类型
* }
* interface Person {
* dance:()=>void
* sing:(song:string)=>void
* }
* interface Person{
* sing:(song:string)=>void
* dance()=>void
* }行为
* 属性
* let yn:Person = {
* sing:(song:string)=>{
* console.log("我赖床是大杯女主",song)
* }}
*
7.联合类型
*联合类型
* let judge : string | number = 100;
*
* 性别:男 女 保密
* let gender:'男' | '女' | '保密' = "男"
*
* 枚举 约定变量只能在一组数据范围内选择 提高可维护性
* 声明枚举类型 使用枚举数据
* enum ThemeColor{
* Red = "#ff0f29"
* }
* let color:ThemeColor = ThemeColor.Red
// 需求:定义一个变量,存放【年终考试评价】
// 考试评价:可能是具体的分数,也可能是A、B、C、D等级
// let judge: number | string = 100
// judge = 'A+'
// judge = '优秀'
// judge = '不错,继续努力'
// console.log('年终考试评价', judge)
// 联合类型还可以将变量值,约定在一组数据范围内进行选择
// 性别:男 女 保密
let gender: 'man' | 'woman' | 'secret' = 'secret'
struct Index {
message: string = 'Hello World';
build() {
Row() {
Column() {
Text(this.message)
.fontSize(50)
.fontWeight(FontWeight.Bold)
}
.width('100%')
}
.height('100%')
}
}
5.枚举
// 需求:利用枚举类型,给变量设置主色
// 取色范围:
// 红色 '#ff0f29'
// 橙色 '#ff7100'
// 绿色 '#30b30e'
// 1. 定义枚举 (定义常量列表)
enum ThemeColor {
Red = '#ff0f29',
Orange = '#ff7100',
Green = '#30b30e'
}
// 2. 给变量设定枚举类型
let color: ThemeColor = ThemeColor.Orange
console.log('color', color)
struct Index {
message: string = 'Hello World';
build() {
Row() {
Column() {
Text(this.message)
.fontSize(50)
.fontWeight(FontWeight.Bold)
}
.width('100%')
}
.height('100%')
}
}
四、基本页面绘制语法
1.文本(颜色,字体大小,颜色,粗细)
1.文本容器
// 以前学基础 → 写代码的位置(页面顶部)
struct Index {
message: string = '学鸿蒙';
// 构建 → 界面
build() {
// 布局思路:先排版,再内容
Column() {
// 内容
Text('小说简介')
Row() {
Text('都市')
Text('生活')
Text('情感')
Text('男频')
}
}
}
}
Index {
message: string = '学鸿蒙';
build() {
// 1. 演示文字颜色 (枚举 + 十六进制)
// Column() {
// Text('吕布')
// .fontSize(30)
// .fontColor('#df3c50')
// // .fontColor(Color.Orange)
// }
// 2. 综合练习:今日头条置顶新闻
// 思路:排版 → 内容 → 美化
Column() {
Text('学鸿蒙~')
.width('100%')
.height(40)
.fontSize(24)
Row() {
Text('置顶 ')
.fontColor('#df3c50')
Text('新华社 ')
.fontColor('#a1a1a1')
Text('4680评论')
.fontColor('#a1a1a1')
}
.width('100%')
}
.width('100%')
}
}
struct
Index {
message: string = '你好可可';
build() {
RelativeContainer() {
Text(this.message)
.id('HelloWorld')
.fontSize(30)
.fontWeight(FontWeight.Bold)
.fontColor(Color.Green)
.alignRules({
center: { anchor: '__container__', align: VerticalAlign.Center },
middle: { anchor: '__container__', align: HorizontalAlign.Center }
})
}
.height('100%')
.width('100%')
}
}
struct
2.图片
// 网络图片地址:
// https://www.itheima.com/images/logo.png
struct Index {
message: string = '学鸿蒙来';
build() {
// 1. 网络图片加载 Image('网图地址')
// Column() {
// Image('https://www.itheima.com/images/logo.png')
// .height(50)
// }
// 2. 本地图片加载 Image( $r('app.media.文件名') )
Column() {
Image($r('app.media.product'))
.width(200)
Text('耐克龙年限定款!!!')
.width(200)
Row() {
Image($r('app.media.avatar'))
.width(20)
Text('令人脱发的代码')
}
.width(200)
}
}
}
3.输入框
Index {
message: string = '学鸿蒙';
build() {
// 控制组件间的距离,可以给 Column 设置 { space: 间隙大小 }
Column({ space: 15 }) {
TextInput({
placeholder: '请输入用户名'
})
TextInput({
placeholder: '请输入密码'
}).type(InputType.Password)
Button('登录')
.width(200)
}
}
}
struct
Index {
message: string = '学鸿蒙';
build() {
// 构建界面核心思路:
// 1. 排版(思考布局)
// 2. 内容 (基础组件)
// 3. 美化 (属性方法)
Column({ space: 15 }) {
Image($r('app.media.huawei'))
.width(50)
TextInput({
placeholder: '请输入用户名'
})
TextInput({
placeholder: '请输入密码'
}).type(InputType.Password)
Button('登录')
.width('100%')
Row({ space: 15 }) {
Text('前往注册')
Text('忘记密码')
}
}
.width('100%')
.padding(20)
}
}
struct
4.交叉轴对齐方式
//alignItems(HorizontalAlign) 主轴比如说横向布局 那就是横向说主轴 交叉轴就是竖轴
//align item 交叉轴是在水平方向 horizontal align 交叉轴在垂直方向vertical align
// 图片 本地图片和在线图片 Image('https://developer.huawei.com/allianceCmsResource/resource/HUAWEI_Developer_VUE/images/080662.png')
struct Index {
message: string = '你好可可';
build() {//33集 个人中心 顶部导航
//Column 交叉轴方向对齐 水平往右
//alignItems(HorizontalAlign)
Column({space:10}){//svg可以方法缩小不失真
Text('当前盒子1').width(100).height(100).backgroundColor(Color.Brown)
Text('当前盒子1').width(100).height(100).backgroundColor(Color.Brown)
Text('当前盒子1').width(100).height(100).backgroundColor(Color.Brown)
}.alignItems(HorizontalAlign.Start).width('100%').height('100%').backgroundColor(Color.Pink)
}
}
// 图片 本地图片和在线图片 Image('https://developer.huawei.com/allianceCmsResource/resource/HUAWEI_Developer_VUE/images/080662.png')
struct Index {
message: string = '你好可可';
build() {//33集 个人中心 顶部导航
//Column 交叉轴方向对齐 水平往右
//alignItems(HorizontalAlign)
Row({space:10}){//svg可以方法缩小不失真
Text('当前盒子1').width(100).height(100).backgroundColor(Color.Brown)
Text('当前盒子1').width(100).height(100).backgroundColor(Color.Brown)
Text('当前盒子1').width(100).height(100).backgroundColor(Color.Brown)
}.width('100%').height('100%').backgroundColor(Color.Pink).alignItems(VerticalAlign.Top)
}1
}
布局练习
// 图片 本地图片和在线图片 Image('https://developer.huawei.com/allianceCmsResource/resource/HUAWEI_Developer_VUE/images/080662.png')
struct Index {
build() {
Column() {
Row({space:10}){
Column({space:10}){
Text('玩一玩').fontSize(20).fontWeight(FontWeight.Bold)
Text('签到兑礼 | 超多大奖 超好玩').fontColor('#999').fontSize(12)
}.alignItems(HorizontalAlign.Start)
Row(){
Image($r("app.media.tree")).width(50)
Image($r('app.media.enter_icon')).width(30)
}
}.width('100%').height(100).borderRadius(5).margin({bottom:10}).backgroundColor(Color.White).justifyContent(FlexAlign.SpaceBetween).padding({left:10,right:10})
Row({space:10}){
Column({space:10}){
Text('玩一玩').fontSize(20).fontWeight(FontWeight.Bold)
Text('签到兑礼 | 超多大奖 超好玩').fontColor('#999').fontSize(12)
}.alignItems(HorizontalAlign.Start)
Row(){
Image($r("app.media.tree")).width(50)
Image($r('app.media.enter_icon')).width(30)
}
}.width('100%').height(100).borderRadius(5).margin({bottom:10}).backgroundColor(Color.White).justifyContent(FlexAlign.SpaceBetween).padding({left:10,right:10})
}.padding({left:10,right:10}).backgroundColor('#ccc').width('100%').height('100%')
}
}
5.自适应伸缩
// 图片 本地图片和在线图片 Image('https://developer.huawei.com/allianceCmsResource/resource/HUAWEI_Developer_VUE/images/080662.png')
//自适应伸缩 设置layoutWeight属性的子元素与兄弟元素 会按照权重进行分配主轴的空间
//语法 .layoutWeight 数字
struct Index {
build() {
Column() {
Row(){
Text('张三').layoutWeight(1).backgroundColor(Color.Black).height(100)
Text('李四').layoutWeight(2).backgroundColor(Color.Yellow).height(100)
Text('王五').layoutWeight(2).height(100)
}
}.padding({left:10,right:10}).backgroundColor('#ccc').width('100%').height('100%')
}
}
// 图片 本地图片和在线图片 Image('https://developer.huawei.com/allianceCmsResource/resource/HUAWEI_Developer_VUE/images/080662.png')
//自适应伸缩 设置layoutWeight属性的子元素与兄弟元素 会按照权重进行分配主轴的空间
//语法 .layoutWeight 数字
struct Index {
build() {
Column() {
Row(){
Text('张三').layoutWeight(1).backgroundColor(Color.Black).height(100)
Text('李四').layoutWeight(2).backgroundColor(Color.Yellow).height(100)
Text('王五').layoutWeight(2).height(100)
}
Row(){
Text('张三').backgroundColor(Color.Black).height(100)
Text('李四').layoutWeight(1).backgroundColor(Color.Yellow).height(100)
Text('王五').height(100)
}
}.padding({left:10,right:10}).backgroundColor('#ccc').width('100%').height('100%')
}
}
练习
// 图片 本地图片和在线图片 Image('https://developer.huawei.com/allianceCmsResource/resource/HUAWEI_Developer_VUE/images/080662.png')
//自适应伸缩 设置layoutWeight属性的子元素与兄弟元素 会按照权重进行分配主轴的空间
//语法 .layoutWeight 数字
struct Index {
build() {
Column() {
Column(){
Text('').height(300).backgroundImage($r('app.media.menghuan')).width('100%').backgroundImageSize(ImageSize.Cover).borderRadius({topLeft:5,topRight:5})
Text('今晚就吃这个 | 每日艺术品分享No.16').fontSize(25).fontWeight(FontWeight.Bold).margin({
top:10,
bottom:10
})
Row(){
Row({space:5}){
Image($r('app.media.background')).borderRadius(16).width(32).height(32)
Text('林可可插画师分享素材')
}
Row({space:5}){
Image($r('app.media.dianzan')).width(22).height(22).fillColor('#999')
Text('2300')
}
}.justifyContent(FlexAlign.SpaceBetween).width('100%').padding({left:5,right:5})
}
}.padding({left:5,right:5}).width('100%').height('100%')
}
}
京东练习
// 图片 本地图片和在线图片 Image('https://developer.huawei.com/allianceCmsResource/resource/HUAWEI_Developer_VUE/images/080662.png')
//自适应伸缩 设置layoutWeight属性的子元素与兄弟元素 会按照权重进行分配主轴的空间
//语法 .layoutWeight 数字
struct Index {
build() {
Column() {
Row(){
Image($r('app.media.jd_cancel')).width(20)
Text('帮助')
}.justifyContent(FlexAlign.SpaceBetween).width('100%')
Image($r('app.media.jd_logo')).width(250).height(250)
Row(){
Text('国家/地址').fontColor('#666').layoutWeight(1)
Text('中国(+86)').fontColor('#666').margin({right:5})
Image($r('app.media.jd_right')).width(20)
}.width('100%').backgroundColor(Color.White).height(40).margin(20).padding({left:10,right:10}).borderRadius(20)
TextInput({
placeholder:'请输入手机号'
}).height(40).borderRadius(20).backgroundColor(Color.White).padding({left:10}).placeholderColor('#666')
Row(){
Checkbox().width(10)
Text(){
Span('我已经阅读并同意')
Span('《京东隐私协议》').fontColor('#3274f6')
Span('《京东用户服务协议》').fontColor('#3274f6')
Span('未注册的手机号将自动创建京东账号')
}.fontSize(12).fontColor('#666').lineHeight(20)
}.alignItems(VerticalAlign.Top).margin({top:20,bottom:20})
Button('登录').width('100%').backgroundColor('#bf2838')
Row({space:25}){
Text('新用户注册').fontSize(14).fontColor('#666')
Text('账号密码无法登陆').fontSize(14).fontColor('#666')
Text('无法登陆').fontSize(14).fontColor('#666')
}.margin({top:15})
Blank()
Column(){
Text('其他方式登录').fontColor('#666').fontSize(14).height(22).margin({bottom:28})
Row(){//blank填充组件
Image($r('app.media.jd_huawei')).width(34)
Image($r('app.media.jd_QQ')).width(34).fillColor('##5c9adb')
Image($r('app.media.jd_weibo')).width(34).fillColor('#c54842')
Image($r('app.media.jd_wechat')).width(34).fillColor('#56a44a')
}.margin({bottom:28}).justifyContent(FlexAlign.SpaceAround).width('100%')
}.width('100%')
}.padding(10).width('100%').height('100%').backgroundImage($r('app.media.jd_login_bg')).backgroundImageSize(ImageSize.Cover)
}
}
6.弹性布局
// 图片 本地图片和在线图片 Image('https://developer.huawei.com/allianceCmsResource/resource/HUAWEI_Developer_VUE/images/080662.png')
//自适应伸缩 设置layoutWeight属性的子元素与兄弟元素 会按照权重进行分配主轴的空间
// ColumnReverse 反转
struct Index {
build() {
Flex({
direction:FlexDirection.Column,
justifyContent:FlexAlign.SpaceAround
}){//flex默认水平往右,交叉轴往下
Text().width(80).height(80).backgroundColor(Color.Pink).border({width:1,color:Color.Black})
Text().width(80).height(80).backgroundColor(Color.Pink).border({width:1,color:Color.Black})
Text().width(80).height(80).backgroundColor(Color.Pink).border({width:1,color:Color.Black})
}.width('100%').height(600).backgroundColor(Color.Gray)
}
}
// 图片 本地图片和在线图片 Image('https://developer.huawei.com/allianceCmsResource/resource/HUAWEI_Developer_VUE/images/080662.png')
//自适应伸缩 设置layoutWeight属性的子元素与兄弟元素 会按照权重进行分配主轴的空间
// ColumnReverse 反转 39
struct Index {
build() {
// Flex({
// direction:FlexDirection.Column,
// justifyContent:FlexAlign.SpaceAround,
// alignItems:ItemAlign.Stretch
// }){//flex默认水平往右,交叉轴往下
// Text().width(80).height(80).backgroundColor(Color.Pink).border({width:1,color:Color.Black})
// Text().width(80).height(80).backgroundColor(Color.Pink).border({width:1,color:Color.Black})
// Text().width(80).height(80).backgroundColor(Color.Pink).border({width:1,color:Color.Black})
// }.width('100%').height(600).backgroundColor(Color.Gray)
Column(){
Text('阶段选择')
Flex({
wrap:FlexWrap.NoWrap
}){
Text('ArkUl').padding(10).backgroundColor(Color.Gray).width(100).margin({right:10})
Text('ArkUl').padding(10).backgroundColor(Color.Gray).width(100).margin({right:10})
Text('ArkUl').padding(10).backgroundColor(Color.Gray).width(100).margin({right:10})
Text('ArkUl').padding(10).backgroundColor(Color.Gray).width(100).margin({right:10})
Text('ArkUl').padding(10).backgroundColor(Color.Gray).width(100).margin({right:10})
Text('ArkUl').padding(10).backgroundColor(Color.Gray).width(100).margin({right:10})
Text('ArkUl').padding(10).backgroundColor(Color.Gray).width(100).margin({right:10})
Text('ArkUl').padding(10).backgroundColor(Color.Gray).width(100).margin({right:10})
Text('ArkUl').padding(10).backgroundColor(Color.Gray).width(100).margin({right:10})
Text('ArkUl').padding(10).backgroundColor(Color.Gray).width(100).margin({right:10})
}
}.width('100%').height('100%')
}
}
// 图片 本地图片和在线图片 Image('https://developer.huawei.com/allianceCmsResource/resource/HUAWEI_Developer_VUE/images/080662.png')
//自适应伸缩 设置layoutWeight属性的子元素与兄弟元素 会按照权重进行分配主轴的空间
// ColumnReverse 反转 39
struct Index {
build() {
// Flex({
// direction:FlexDirection.Column,
// justifyContent:FlexAlign.SpaceAround,
// alignItems:ItemAlign.Stretch
// }){//flex默认水平往右,交叉轴往下
// Text().width(80).height(80).backgroundColor(Color.Pink).border({width:1,color:Color.Black})
// Text().width(80).height(80).backgroundColor(Color.Pink).border({width:1,color:Color.Black})
// Text().width(80).height(80).backgroundColor(Color.Pink).border({width:1,color:Color.Black})
// }.width('100%').height(600).backgroundColor(Color.Gray)
Column(){
Text('阶段选择')
Flex({
wrap:FlexWrap.Wrap
}){
Text('ArkUl').padding(10).backgroundColor(Color.Gray).width(100).margin({right:10})
Text('ArkUl').padding(10).backgroundColor(Color.Gray).width(100).margin({right:10})
Text('ArkUl').padding(10).backgroundColor(Color.Gray).width(100).margin({right:10})
Text('ArkUl').padding(10).backgroundColor(Color.Gray).width(100).margin({right:10})
Text('ArkUl').padding(10).backgroundColor(Color.Gray).width(100).margin({right:10})
Text('ArkUl').padding(10).backgroundColor(Color.Gray).width(100).margin({right:10})
Text('ArkUl').padding(10).backgroundColor(Color.Gray).width(100).margin({right:10})
Text('ArkUl').padding(10).backgroundColor(Color.Gray).width(100).margin({right:10})
Text('ArkUl').padding(10).backgroundColor(Color.Gray).width(100).margin({right:10})
Text('ArkUl').padding(10).backgroundColor(Color.Gray).width(100).margin({right:10})
}
}.width('100%').height('100%')
}
}
6.绝对定位和层级
// 图片 本地图片和在线图片 Image('https://developer.huawei.com/allianceCmsResource/resource/HUAWEI_Developer_VUE/images/080662.png')
//自适应伸缩 设置layoutWeight属性的子元素与兄弟元素 会按照权重进行分配主轴的空间
// Position
struct Index {
build() {
Column(){
Text('大儿子').width(80).height(80).backgroundColor(Color.Gray)
Text('2儿子').width(80).height(80).backgroundColor(Color.Green).position({x:100,y:50}).zIndex(10)
Text('3儿子').width(80).height(80).backgroundColor(Color.Yellow)
}.width('100%').height('100%')
}
}
练习
思路分析:
- 整体:从上往下布局 Column
- 局部:先图片、再Row(图 + 文本)
- 细节:VIP(定位、圆角)
Text内文本对齐方式:
.textAlign(TextAlign.Center)
// 图片 本地图片和在线图片 Image('https://developer.huawei.com/allianceCmsResource/resource/HUAWEI_Developer_VUE/images/080662.png')
//自适应伸缩 设置layoutWeight属性的子元素与兄弟元素 会按照权重进行分配主轴的空间
// Position
struct Index {
build() {
Column(){
Column(){
//定位vip
Text('VIP').textAlign(TextAlign.Center).position({x:0,y:0}).zIndex(100).width(40).height(20).backgroundColor(Color.Orange).fontColor(Color.White).borderRadius({topLeft:10,bottomRight:10}).border({
width:2,color:'#fbe7a3'//.padding({left:5})
}).fontStyle(FontStyle.Italic).fontSize(14).fontWeight(700)
Image($r('app.media.position_moco')).width('100%').height(210).borderRadius({topLeft:10,topRight:10})
Row(){
Image($r('app.media.position_earphone')).width(20).borderRadius(10).backgroundColor('#55b7f4').padding(3).fillColor(Color.White)
Text('飞狗MOCO').margin({left:10})
}.width('100%').margin(10)
}.width(160).height(240)
}.width('100%').height('100%')
}
}
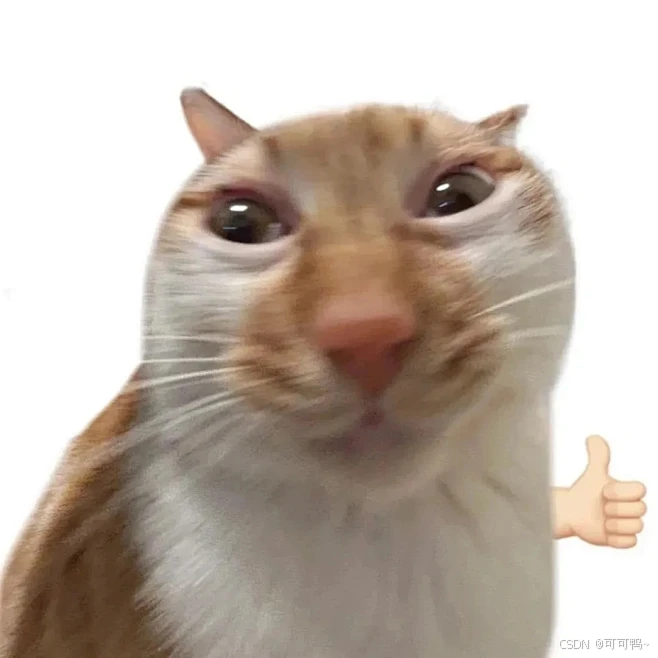
原文地址:https://blog.csdn.net/qq_43547255/article/details/144903584
免责声明:本站文章内容转载自网络资源,如本站内容侵犯了原著者的合法权益,可联系本站删除。更多内容请关注自学内容网(zxcms.com)!