Java、JavaWeb、数据库-图书管理系统
这一章主要是把上一章写在网页里的java 代码从网页中分离出来,放在专门的servlet类中。每一个servlet类对应一个数据库的表。
规范性问题:
1、dao包存放有关数据库的信息:BaseDao包就放数据库加载驱动和增删改和关闭资源;而其他的每一个表名对应一个相应名字的dao包,写数据库的增删改查操作;
2、servlet包专存放和网页有关的代码,把网页传来的参数进行接受,同时调用相应名字的dao类中的方法,返回到相应的页面。
3、untity包专门放对应表格的实体类
4、test包专门放测试的方法,单元测试等。
整合前面的的学生管理系统,重构一个图书管理系统,要求规范性,且网页中不在出现java代码,都放在servlet类中
在写代码前先建好相应的包,并且导入数据库的jar包,web的jar包,还有tomcat的配置,以及在数据库编辑软件navicat建好相应的数据表。
图书管理系统的运行截图:左上方两个表在数据库建好
登录界面展示:
注册界面展示:
各种功能展示: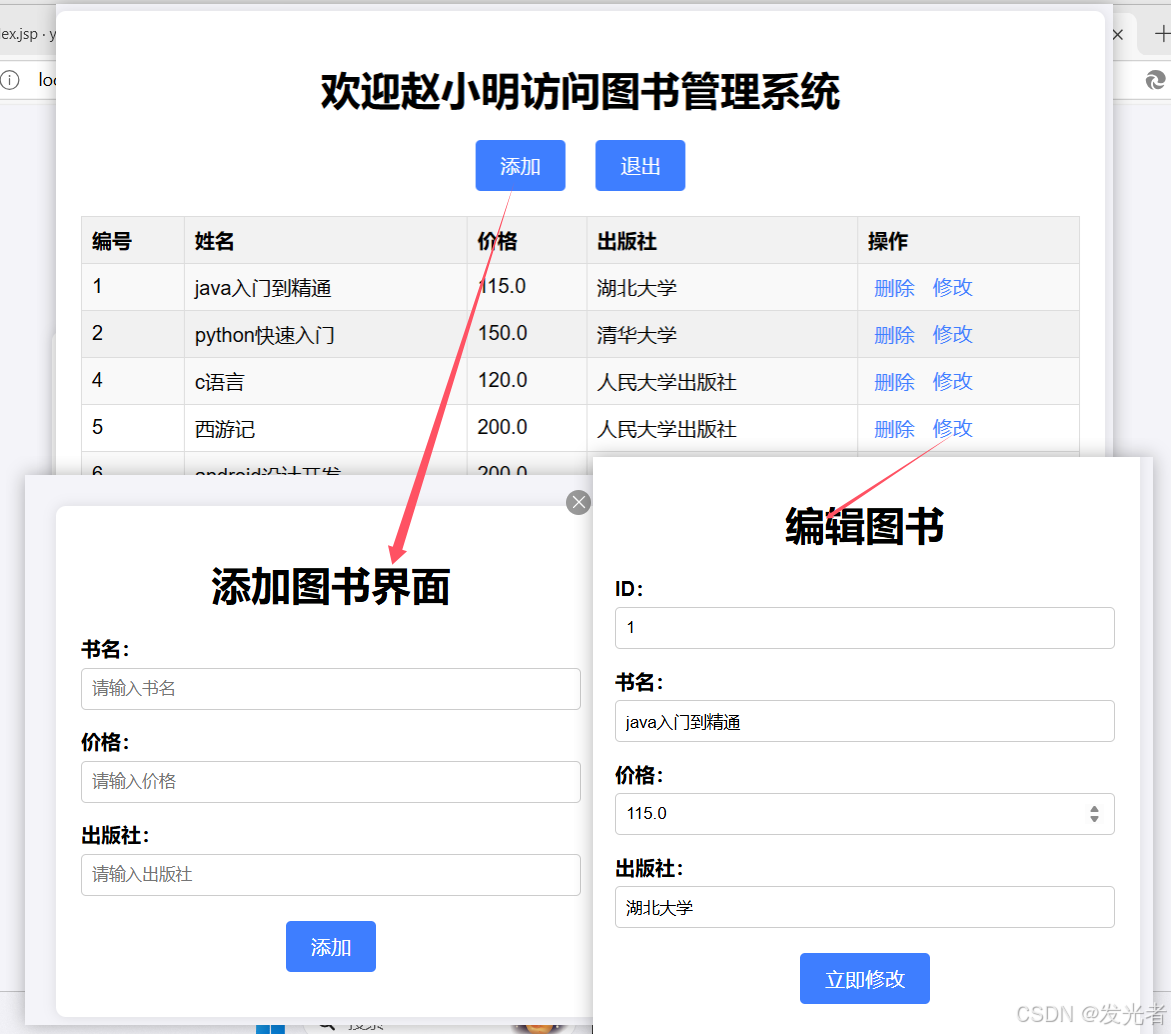
一、代码:
BaseDao:
package com.zyl.dao; import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; /** * @className: BaseDao * @author: Zyl * @date: 2024/12/3 16:49 * @Version: 1.0 * @description: */ public class BaseDao { Connection connection= null; PreparedStatement ps=null; ResultSet resultSet = null; String url = "jdbc:mysql://localhost:3306/jdbctest"; String username = "root"; String password = "root"; String DriverName="com.mysql.jdbc.Driver"; //加载驱动,获取连接,连接数据库 public void connection()throws Exception{ Class.forName(DriverName); connection = DriverManager.getConnection(url, username, password); } //针对增删改的方法,没有查询的功能 public int edit(String sql,Object... params) { try { connection(); ps = connection.prepareStatement(sql); for (int i = 0; i < params.length; i++) { ps.setObject(i + 1, params[i]); } int row = ps.executeUpdate(); return row; } catch (Exception e) { throw new RuntimeException(e); } finally { closeAll(); } } //关闭所有的资源 public void closeAll(){ try { if(resultSet!=null){ resultSet.close(); } if(ps!=null){ ps.close(); } if(connection!=null){ connection.close(); } }catch (Exception e){ throw new RuntimeException(e); } } }
BookDao:
package com.zyl.dao; import com.zyl.untity.Book; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.util.ArrayList; /** * @className: BookDao * @author: Zyl * @date: 2024/12/6 10 * @Version: 1.0 * @description: */ public class BookDao extends BaseDao{ //查询所有的图书 public ArrayList<Book> selectAll(){ ArrayList<Book> list=new ArrayList<Book>(); try { connection(); String sql="select * from tbl_book"; ps = connection.prepareStatement(sql); resultSet = ps.executeQuery(); while (resultSet.next()){ int id=resultSet.getInt("id"); String name=resultSet.getString("name"); double price=resultSet.getDouble("price"); String publisher=resultSet.getString("publisher"); Book book =new Book(); book.setId(id); book.setName(name); book.setPrice(price); book.setPublisher(publisher); list.add(book); } return list; } catch (Exception e) { throw new RuntimeException(e); } finally { closeAll(); } } public int delete(int id){ String sql = "delete from tbl_book where id=?"; //d调用父类BaseDao 的edit()方法 增删改操作;;;;;; int edit = edit(sql,id); return edit; } public Book findById(int id){ Book book =null; try { String sql = "select * from tbl_book where id=?"; connection(); ps = connection.prepareStatement(sql); ps.setInt(1,id); resultSet = ps.executeQuery(); while (resultSet.next()){ int id1=resultSet.getInt("id"); String name=resultSet.getString("name"); double price=resultSet.getDouble("price"); String publisher=resultSet.getString("publisher"); book=new Book(id1,name,price,publisher); } return book; } catch (Exception e) { throw new RuntimeException(e); } finally { closeAll(); } } public int update(int id,String name,double price,String publisher ){ String sql = "update tbl_book set name=?,price=?,publisher=?where id=?"; //d调用父类BaseDao 的edit()方法 增删改操作;;;;;; int edit = edit(sql,name,price,publisher,id); return edit; } public int insert(String name,double price,String publisher ){ String sql = "insert into tbl_book(name,price,publisher) values(?,?,?)"; //d调用父类BaseDao 的edit()方法 增删改操作;;;;;; int edit = edit(sql,name,price,publisher); return edit; } }
UserDao:
package com.zyl.dao; import com.zyl.untity.User; /** * @className: UserDao * @author: Zyl * @date: 2024/12/6 10:15 * @Version: 1.0 * @description: */ public class UserDao extends BaseDao{ public User login(String username, String password){ User user=null; try { String sql="select * from tbl_user where username=? and password=?"; connection(); ps = connection.prepareStatement(sql); ps.setObject(1,username); ps.setObject(2,password); resultSet = ps.executeQuery(); while(resultSet.next()){ int id = resultSet.getInt("id"); String username1 = resultSet.getString("username"); String password1 = resultSet.getString("password"); String realname = resultSet.getString("realname"); int age = resultSet.getInt("age"); // user=new User(username1,id,password1,realname,age); user=new User(); user.setId(id); user.setUsername(username1); user.setAge(age); user.setPassword(password1); user.setRealname(realname); } } catch (Exception e) { throw new RuntimeException(e); } finally { closeAll(); } return user; } public int add(String username, String password){ String sql="insert into tbl_user(username,password) values(?,?)"; int i=edit(sql,username,password); return i; } }
BookServlet:
package com.zyl.servlet; import com.zyl.dao.BookDao; import com.zyl.untity.Book; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; import java.io.UnsupportedEncodingException; import java.util.ArrayList; /** * @className: BookServlet * @author: Zyl * @date: 2024/12/6 10:26 * @Version: 1.0 * @description: */ @WebServlet(urlPatterns = "/book") public class BookServlet extends HttpServlet { private BookDao bookDao = new BookDao(); @Override protected void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { req.setCharacterEncoding("UTF-8"); String method = req.getParameter("method"); if("delete".equals(method)){ delete(req,resp); }else if("getById".equals(method)){ selectById(req,resp); }else if("update".equals(method)){ update(req,resp); }else if("insert".equals(method)){ insert(req,resp); } else{ selectAll(req,resp); } } public void insert(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { //获取参数 String name = req.getParameter("name"); double price = Double.parseDouble(req.getParameter("price")); String publisher = req.getParameter("publisher"); BookDao bookDao = new BookDao(); int i = bookDao.insert(name, price, publisher); if(i>0){ resp.sendRedirect("/book"); } } private void selectById(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { //获取id String id = req.getParameter("id"); //创建对象 BookDao bookDao = new BookDao(); Book b = bookDao.findById(Integer.parseInt(id)); req.setAttribute("book",b); req.getRequestDispatcher("/edit.jsp").forward(req,resp); } public void selectAll(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException{ BookDao bookDao = new BookDao(); ArrayList<Book> books = bookDao.selectAll(); req.setAttribute("books",books); req.getRequestDispatcher("/index.jsp").forward(req,resp); } public void delete(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException{ String id = req.getParameter("id");//获取id BookDao bookDao = new BookDao();//创建对象 int delete = bookDao.delete(Integer.parseInt(id)); if(delete>0){ resp.sendRedirect("/book"); } } public void update(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException{ //获取参数 String id = req.getParameter("id"); String name = req.getParameter("name"); String price = req.getParameter("price"); String publisher = req.getParameter("publisher"); //先判断在创建对象并调用方法 if(id!=null&&!id.equals("")&&name!=null&&!name.equals("")&&price!=null&&!price.equals("")&&publisher!=null&&!publisher.equals("")){ BookDao b=new BookDao(); int i=b.update(Integer.parseInt(id),name, Double.parseDouble(price),publisher); if(i>0){ resp.sendRedirect("/book"); } } } }
UserServlet:
package com.zyl.servlet; import com.zyl.dao.UserDao; import com.zyl.untity.User; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import javax.servlet.http.HttpSession; import java.io.IOException; import java.io.UnsupportedEncodingException; /** * @className: UserServlet * @author: Zyl * @date: 2024/12/6 10:25 * @Version: 1.0 * @description: */ @WebServlet(urlPatterns = "/user") public class UserServlet extends HttpServlet { private UserDao userDao=new UserDao();//对象 @Override public void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { req.setCharacterEncoding("utf-8"); String method = req.getParameter("method"); if ("login".equals(method)) { login(req, resp); } else if ("register".equals(method)) { register(req, resp); } else if ("exit".equals(method)){ exit(req, resp); } } public void exit(HttpServletRequest req, HttpServletResponse resp)throws ServletException, IOException { HttpSession session=req.getSession(); //获取session,判断是否登录,登录了就删除session,并跳转回登录页面 session.removeAttribute("user"); resp.sendRedirect("/login.jsp"); } public void login(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { String username = req.getParameter("username"); String password = req.getParameter("password"); UserDao userDao = new UserDao(); User user = userDao.login(username, password); if (user!= null) { //保存到session会话 req.getSession().setAttribute("user", user); //跳转--成功页面【展示所有图书】 resp.sendRedirect("/book"); } else { //跳转到登录页面 req.setAttribute("error", "<font color='red'>账号或密码错误</font>"); req.getRequestDispatcher("/login.jsp").forward(req, resp);//转发跳转.必须转发跳转 } } public void register(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { // 获取表单数据 String username = req.getParameter("username"); String password = req.getParameter("password"); String confirmPassword = req.getParameter("confirmPassword"); System.out.println(1222); // 初始化消息变量 String message = ""; String messageType = ""; // 检查密码是否一致 if (!password.equals(confirmPassword)) { message = "两次输入的密码不一致,请重新输入。"; messageType = "error-message"; } else { // 创建 UserDao 实例 UserDao userDao = new UserDao(); // 检查用户名和密码是否同时存在 if (userDao.login(username, password) != null) { message = "该用户名和密码已被注册过。"; messageType = "error-message"; } else { // 调用 add 方法添加学生 int result = userDao.add(username, password); if (result > 0) { message = "注册成功!"; messageType = "success-message"; } else { message = "注册失败,请重试。"; messageType = "error-message"; } } } // 将消息存储到请求属性中 req.setAttribute("message", message); req.setAttribute("messageType", messageType); // 转发到 register.jsp 显示消息 req.getRequestDispatcher("/register.jsp").forward(req, resp); } }
二、网页代码:
bookadd.jsp:
<%-- Created by IntelliJ IDEA. User: Date: 2024/12/7 Time: 16:21 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <html> <head> <title>添加图书界面</title> <style> body { font-family: Arial, sans-serif; background-color: #f4f4f9; display: flex; justify-content: center; align-items: center; height: 100vh; margin: 0; } .container { background-color: #fff; padding: 20px; border-radius: 8px; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); width: 80%; max-width: 400px; text-align: center; } .container h1 { margin-bottom: 20px; } .form-group { margin-bottom: 15px; text-align: left; } .form-group label { display: block; margin-bottom: 5px; font-weight: bold; } .form-group input { width: 100%; padding: 8px; box-sizing: border-box; border: 1px solid #ccc; border-radius: 4px; } .button-container { margin-top: 20px; } .button-container button { padding: 10px 20px; border: none; border-radius: 4px; cursor: pointer; background-color: #007bff; color: white; font-size: 16px; } .button-container button:hover { background-color: #0056b3; } .error-message { color: red; margin-bottom: 15px; } .success-message { color: green; margin-bottom: 15px; } </style> </head> <body> <div class="container"> <h1>添加图书界面</h1> <c:if test="${sessionScope.user==null}"> <c:redirect url="/login.jsp"/> </c:if> <form action="/book?method=insert" method="post"> <div class="form-group"> <label for="name">书名:</label> <input type="text" id="name" name="name" placeholder="请输入书名" required/> </div> <div class="form-group"> <label for="price">价格:</label> <input type="number" id="price" name="price" placeholder="请输入价格" step="0.01" required/> </div> <div class="form-group"> <label for="publisher">出版社:</label> <input type="text" id="publisher" name="publisher" placeholder="请输入出版社" required/> </div> <div class="button-container"> <button type="submit">添加</button> </div> </form> </div> </body> </html>
edit.jsp:
<%-- Created by IntelliJ IDEA. User: Date: 2024/12/7 Time: 15:37 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <html> <head> <title>编辑图书</title> <style> body { font-family: Arial, sans-serif; background-color: #f4f4f9; display: flex; justify-content: center; align-items: center; height: 100vh; margin: 0; } .container { background-color: #fff; padding: 20px; border-radius: 8px; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); width: 80%; max-width: 400px; text-align: center; } .container h1 { margin-bottom: 20px; } .form-group { margin-bottom: 15px; text-align: left; } .form-group label { display: block; margin-bottom: 5px; font-weight: bold; } .form-group input { width: 100%; padding: 8px; box-sizing: border-box; border: 1px solid #ccc; border-radius: 4px; } .button-container { margin-top: 20px; } .button-container button { padding: 10px 20px; border: none; border-radius: 4px; cursor: pointer; background-color: #007bff; color: white; font-size: 16px; } .button-container button:hover { background-color: #0056b3; } .error-message { color: red; margin-bottom: 15px; } .success-message { color: green; margin-bottom: 15px; } </style> </head> <body> <div class="container"> <h1>编辑图书</h1> <c:if test="${sessionScope.user==null}"> <c:redirect url="/login.jsp"/> </c:if> <form action="/book?method=update" method="post"> <div class="form-group"> <label for="id">ID:</label> <input type="text" id="id" name="id" value="${book.getId()}" readonly/> </div> <div class="form-group"> <label for="name">书名:</label> <input type="text" id="name" name="name" placeholder="请输入书名" value="${book.getName()}" required/> </div> <div class="form-group"> <label for="price">价格:</label> <input type="number" id="price" name="price" placeholder="请输入价格" value="${book.getPrice()}" step="0.01" required/> </div> <div class="form-group"> <label for="publisher">出版社:</label> <input type="text" id="publisher" name="publisher" placeholder="请输入出版社" value="${book.getPublisher()}" required/> </div> <div class="button-container"> <button type="submit">立即修改</button> </div> </form> </div> </body> </html>
index.jsp:
<%@ page import="com.zyl.untity.User" %><%-- Created by IntelliJ IDEA. User: ${zyl} Date: 2024/12/6 Time: 10:12 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <html> <head> <title>图书首页</title> <style> body { font-family: Arial, sans-serif; background-color: #f4f4f9; display: flex; justify-content: center; align-items: center; height: 100vh; margin: 0; } .container { background-color: #fff; padding: 20px; border-radius: 8px; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); width: 80%; max-width: 800px; text-align: center; } .container h1 { margin-bottom: 20px; } .button-container { margin-bottom: 20px; } .button-container button { margin: 0 10px; padding: 10px 20px; border: none; border-radius: 4px; cursor: pointer; background-color: #007bff; color: white; font-size: 16px; } .button-container button:hover { background-color: #0056b3; } table { width: 100%; border-collapse: collapse; margin-top: 20px; } th, td { border: 1px solid #ddd; padding: 8px; text-align: left; } th { background-color: #f2f2f2; } tr:nth-child(even) { background-color: #f9f9f9; } tr:hover { background-color: #f1f1f1; } a { margin: 0 5px; text-decoration: none; color: #007bff; } a:hover { text-decoration: underline; } </style> </head> <body> <c:if test="${sessionScope.user == null}"> <c:redirect url="/login.jsp"/> </c:if> <div class="container"> <h1>欢迎${sessionScope.user.realname}访问图书管理系统</h1> <div class="button-container"> <button οnclick="location.href='/bookadd.jsp'">添加</button> <button οnclick="location.href='/user?method=exit'">退出</button> </div> <table> <tr> <th>编号</th> <th>姓名</th> <th>价格</th> <th>出版社</th> <th>操作</th> </tr> <c:forEach items="${requestScope.books}" var="b"> <tr> <td>${b.id}</td> <td>${b.name}</td> <td>${b.price}</td> <td>${b.publisher}</td> <td> <a οnclick="del(${b.id})">删除</a> <a href="/book?method=getById&id=${b.id}">修改</a> </td> </tr> </c:forEach> </table> </div> <script> function del(id) { var b = confirm("是否删除该记录?"); if (b) { location.href = "/book?method=delete&id=" + id; } } </script> </body> </html>
login.jsp:
<%-- Created by IntelliJ IDEA. User: Date: 2024/12/6 Time: 10:50 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>登录界面</title> <style> body { font-family: Arial, sans-serif; background-color: #f4f4f9; display: flex; justify-content: center; align-items: center; height: 100vh; margin: 0; } .login-container { background-color: #fff; padding: 20px; border-radius: 8px; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); width: 300px; text-align: center; } .login-container h2 { margin-bottom: 20px; } .login-container input[type="text"], .login-container input[type="password"] { width: calc(100% - 22px); padding: 10px; margin: 10px 0; border: 1px solid #ccc; border-radius: 4px; } .login-container input[type="submit"], .login-container input[type="button"] { width: calc(100% - 22px); padding: 10px; margin: 10px 0; border: none; border-radius: 4px; cursor: pointer; background-color: #007bff; color: white; font-size: 16px; } .login-container input[type="submit"]:hover, .login-container input[type="button"]:hover { background-color: #0056b3; } .error-message { color: red; margin-bottom: 10px; } </style> </head> <body> <div class="login-container"> <h2>登录界面</h2> <div class="error-message">${error}</div> <form action="/user?method=login" method="post"> <input type="text" name="username" placeholder="账号"/><br> <input type="password" name="password" placeholder="密码"/><br> <input type="submit" value="立即登录"/> <input type="button" value="注册" οnclick="location.href='register.jsp'"/> </form> </div> </body> </html>
register.jsp:
<%-- Created by IntelliJ IDEA. User: Date: 2024/12/7 Time: 10:56 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <html> <head> <title>注册界面</title> <style> body { font-family: Arial, sans-serif; background-color: #f4f4f9; display: flex; justify-content: center; align-items: center; height: 100vh; margin: 0; } .container { background-color: #fff; padding: 20px; border-radius: 8px; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); width: 80%; max-width: 400px; text-align: center; } .container h1 { margin-bottom: 20px; } .form-group { margin-bottom: 15px; text-align: left; } .form-group label { display: block; margin-bottom: 5px; font-weight: bold; } .form-group input { width: 100%; padding: 8px; box-sizing: border-box; border: 1px solid #ccc; border-radius: 4px; } .button-container { margin-top: 20px; } .button-container button { padding: 10px 20px; border: none; border-radius: 4px; cursor: pointer; background-color: #007bff; color: white; font-size: 16px; } .button-container button:hover { background-color: #0056b3; } .error-message { color: red; margin-bottom: 15px; } .success-message { color: green; margin-bottom: 15px; } .login-link { margin-top: 15px; font-size: 14px; } .login-link a { color: #007bff; text-decoration: none; } .login-link a:hover { text-decoration: underline; } </style> </head> <body> <div class="container"> <h1>注册界面</h1> <c:if test="${not empty message}"> <div class="${messageType}">${message}</div> </c:if> <form action="/user?method=register" method="post"> <div class="form-group"> <label for="username">账号:</label> <input type="text" id="username" name="username" required> </div> <div class="form-group"> <label for="password">密码:</label> <input type="password" id="password" name="password" required> </div> <div class="form-group"> <label for="confirmPassword">确认密码:</label> <input type="password" id="confirmPassword" name="confirmPassword" required> </div> <div class="button-container"> <button type="submit">注册</button> </div> <div class="login-link"> <a href="login.jsp">已有账号,立即登录</a> </div> </form> </div> </body> </html>
原文地址:https://blog.csdn.net/weixin_64574904/article/details/144315363
免责声明:本站文章内容转载自网络资源,如本站内容侵犯了原著者的合法权益,可联系本站删除。更多内容请关注自学内容网(zxcms.com)!